Get List of Faces Around a Given Vertex#
Synopsis#
Get the list of faces around a given vertex.
Results#
Results:
79
1961
2036
1960
1
These faces are visualized in the figures below.
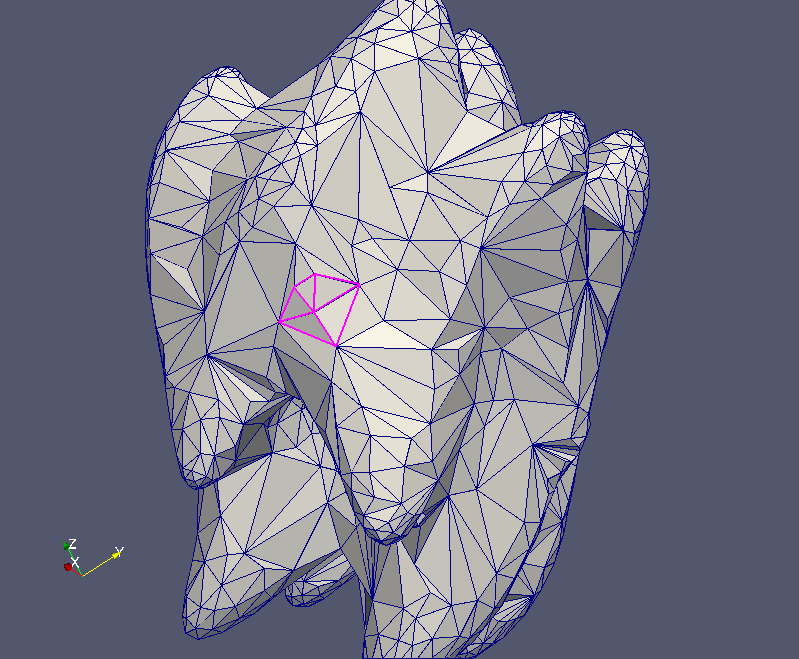
The selected faces around the given vertex are highlighted in pink.#
Interactive view of the selected faces
Code#
C++#
#include "itkMeshFileReader.h"
#include "itkQuadEdgeMesh.h"
int
main(int argc, char * argv[])
{
if (argc != 3)
{
std::cerr << "Usage: " << std::endl;
std::cerr << argv[0];
std::cerr << " <InputFileName> <VertexId>";
std::cerr << std::endl;
return EXIT_FAILURE;
}
constexpr unsigned int Dimension = 3;
using PixelType = double;
using MeshType = itk::QuadEdgeMesh<PixelType, Dimension>;
using ReaderType = itk::MeshFileReader<MeshType>;
auto reader = ReaderType::New();
reader->SetFileName(argv[1]);
try
{
reader->Update();
}
catch (const itk::ExceptionObject & e)
{
std::cerr << e.what() << std::endl;
return EXIT_FAILURE;
}
MeshType::Pointer mesh = reader->GetOutput();
MeshType::PointIdentifier id = std::stoi(argv[2]);
MeshType::QEType * qe = mesh->FindEdge(id);
MeshType::QEType * temp = qe;
do
{
std::cout << temp->GetLeft() << std::endl;
temp = temp->GetOnext();
} while (qe != temp);
return EXIT_SUCCESS;
}
Classes demonstrated#
-
template<typename TPixel, unsigned int VDimension, typename TTraits = QuadEdgeMeshTraits<TPixel, VDimension, bool, bool>>
class QuadEdgeMesh : public itk::Mesh<TPixel, VDimension, TTraits> Mesh class for 2D manifolds embedded in ND space.
This implementation was contributed as a paper to the Insight Journal
https://www.insight-journal.org/browse/publication/122- Author
Alexandre Gouaillard, Leonardo Florez-Valencia, Eric Boix