Get Image Size#
Synopsis#
Get the size of a itk::Image
Results#
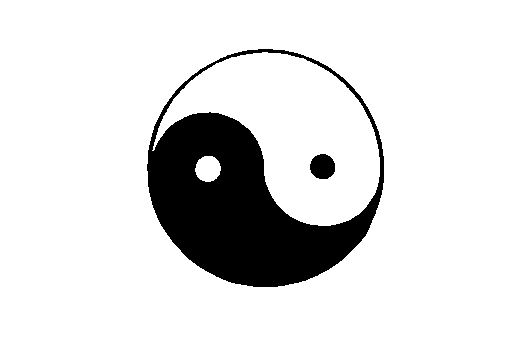
Input Image#
- Output::
[512, 342]
Code#
C++#
#include "itkImage.h"
#include "itkImageFileReader.h"
int
main(int argc, char * argv[])
{
// Verify command line arguments
if (argc < 2)
{
std::cerr << "Usage: " << std::endl;
std::cerr << argv[0] << " inputImageFile" << std::endl;
return EXIT_FAILURE;
}
using PixelType = unsigned char;
using ImageType = itk::Image<PixelType, 2>;
ImageType::Pointer image = itk::ReadImage<ImageType>(argv[1]);
ImageType::RegionType region = image->GetLargestPossibleRegion();
ImageType::SizeType size = region.GetSize();
std::cout << size << std::endl;
// An example image had w = 200 and h = 100
// (it is wider than it is tall). The above output
// 200 x 100
// so w = GetSize()[0]
// and h = GetSize()[1]
// A pixel inside the region
ImageType::IndexType indexInside;
indexInside[0] = 150;
indexInside[1] = 50;
std::cout << region.IsInside(indexInside) << std::endl;
// A pixel outside the region
ImageType::IndexType indexOutside;
indexOutside[0] = 50;
indexOutside[1] = 150;
std::cout << region.IsInside(indexOutside) << std::endl;
// This means that the [0] component of the index is referencing the
// left to right (x) value and the [1] component of Index is referencing
// the top to bottom (y) value
return EXIT_SUCCESS;
}
Python#
#!/usr/bin/env python
import numpy as np
import itk
import argparse
parser = argparse.ArgumentParser(description="Get Image Size.")
parser.add_argument("input_image")
args = parser.parse_args()
image = itk.imread(args.input_image, itk.UC)
region = image.GetLargestPossibleRegion()
size = region.GetSize()
print(size)
# Equivalently
size = itk.size(image)
print(size)
# Corresponds to the NumPy ndarray shape. Note that the ordering is reversed.
print(np.asarray(image).shape)
# An example image had w = 200 and h = 100
# (it is wider than it is tall). The above output
# 200 x 100
# so w = GetSize()[0]
# and h = GetSize()[1]
# A pixel inside the region
indexInside = itk.Index[2]()
indexInside[0] = 150
indexInside[1] = 50
print(region.IsInside(indexInside))
# A pixel outside the region
indexOutside = itk.Index[2]()
indexOutside[0] = 50
indexOutside[1] = 150
print(region.IsInside(indexOutside))
# This means that the [0] component of the index is referencing the
# left to right (x) value and the [1] component of Index is referencing
# the top to bottom (y) value
Classes demonstrated#
-
template<unsigned int VDimension = 2>
struct Size Represent a n-dimensional size (bounds) of a n-dimensional image.
Size is a templated class to represent multi-dimensional array bounds, i.e. (I,J,K,…). Size is templated over teh dimension of the bounds. ITK assumes the first element of a size (bounds) is the fastest moving index.
For efficiency, Size does not define a default constructor, a copy constructor, or an operator=. We rely on the compiler to provide efficient bitwise copies.
Size is an “aggregate” class. Its data is public (m_InternalArray) allowing for fast and convenient instantiations/assignments.
The following syntax for assigning an aggregate type like this is allowed/suggested:
Size<3> var{{ 256, 256, 20 }}; // Also prevent narrowing conversions Size<3> var = {{ 256, 256, 20 }};
The doubled braces {{ and }} are required to prevent gcc -Wall (and perhaps other compilers) from complaining about a partly bracketed initializer.
As an aggregate type that is intended to provide highest performance characteristics, this class is not appropriate to inherit from, so setting this struct as final.
- See
Index
- ITK Sphinx Examples: