Convolve Image With Kernel#
Synopsis#
Convolve an image with a kernel.
Results#
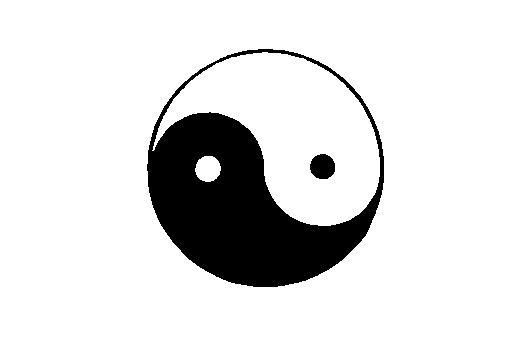
Input image.#
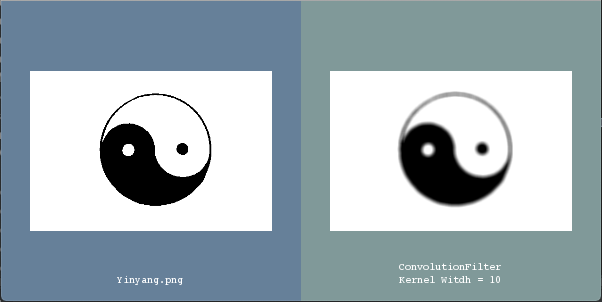
Output In VTK Window#
Code#
C++#
#include "itkImage.h"
#include "itkImageFileReader.h"
#include "itkConvolutionImageFilter.h"
#include "itkImageRegionIterator.h"
#ifdef ENABLE_QUICKVIEW
# include "QuickView.h"
#endif
using ImageType = itk::Image<float, 2>;
static void
CreateKernel(ImageType::Pointer kernel, unsigned int width);
int
main(int argc, char * argv[])
{
// Verify command line arguments
if (argc < 2)
{
std::cerr << "Usage: ";
std::cerr << argv[0] << "inputImageFile [width]" << std::endl;
return EXIT_FAILURE;
}
// Parse command line arguments
unsigned int width = 3;
if (argc > 2)
{
width = std::stoi(argv[2]);
}
auto kernel = ImageType::New();
CreateKernel(kernel, width);
const auto input = itk::ReadImage<ImageType>(argv[1]);
// Convolve image with kernel.
using FilterType = itk::ConvolutionImageFilter<ImageType>;
auto convolutionFilter = FilterType::New();
convolutionFilter->SetInput(input);
#if ITK_VERSION_MAJOR >= 4
convolutionFilter->SetKernelImage(kernel);
#else
convolutionFilter->SetImageKernelInput(kernel);
#endif
#ifdef ENABLE_QUICKVIEW
QuickView viewer;
viewer.AddImage<ImageType>(input, true, itksys::SystemTools::GetFilenameName(argv[1]));
std::stringstream desc;
desc << "ConvolutionFilter\n"
<< "Kernel Witdh = " << width;
viewer.AddImage<ImageType>(convolutionFilter->GetOutput(), true, desc.str());
viewer.Visualize();
#endif
return EXIT_SUCCESS;
}
void
CreateKernel(ImageType::Pointer kernel, unsigned int width)
{
ImageType::IndexType start;
start.Fill(0);
ImageType::SizeType size;
size.Fill(width);
ImageType::RegionType region;
region.SetSize(size);
region.SetIndex(start);
kernel->SetRegions(region);
kernel->Allocate();
itk::ImageRegionIterator<ImageType> imageIterator(kernel, region);
while (!imageIterator.IsAtEnd())
{
// imageIterator.Set(255);
imageIterator.Set(1);
++imageIterator;
}
}
Classes demonstrated#
-
template<typename TInputImage, typename TKernelImage = TInputImage, typename TOutputImage = TInputImage>
class ConvolutionImageFilter : public itk::ConvolutionImageFilterBase<TInputImage, TKernelImage, TOutputImage> Convolve a given image with an arbitrary image kernel.
This filter operates by centering the flipped kernel at each pixel in the image and computing the inner product between pixel values in the image and pixel values in the kernel. The center of the kernel is defined as
where
is the index and
is the size of the largest possible region of the kernel image. For kernels with odd sizes in all dimensions, this corresponds to the center pixel. If a dimension of the kernel image has an even size, then the center index of the kernel in that dimension will be the largest integral index that is less than the continuous index of the image center.
The kernel can optionally be normalized to sum to 1 using NormalizeOn(). Normalization is off by default.
This code was contributed in the Insight Journal paper:
- Warning
This filter ignores the spacing, origin, and orientation of the kernel image and treats them as identical to those in the input image.
“Image Kernel Convolution” by Tustison N., Gee J. http://insight-journal.org/browse/publication/208
- Author
Nicholas J. Tustison
- Author
James C. Gee
- ITK Sphinx Examples: