Iterate Region in Image With Access to Current Index With Write Access#
Synopsis#
Iterate over a region of an image with efficient access to the current index (with write access).
Results#
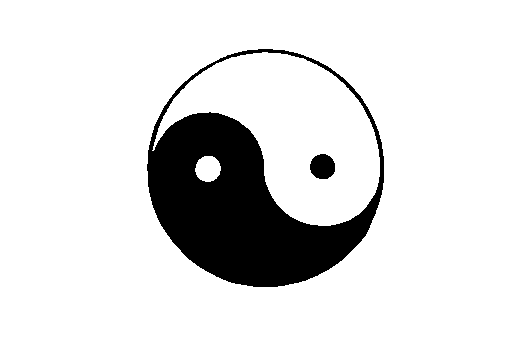
Yinyang.png#
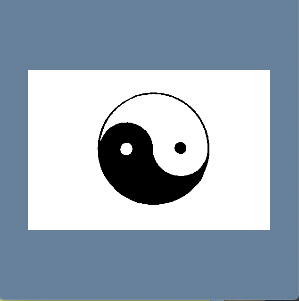
Yinyang.png In VTK Window With Index Access#
Output:
Indices:
Index: [0, 0] value: ?
Index: [1, 0] value: ?
Index: [2, 0] value: ?
Index: [3, 0] value: ?
Index: [4, 0] value: ?
Index: [0, 1] value: ?
Index: [1, 1] value: ?
Index: [2, 1] value: ?
Index: [3, 1] value: ?
Index: [4, 1] value: ?
Index: [0, 2] value: ?
Index: [1, 2] value: ?
Index: [2, 2] value: ?
Index: [3, 2] value: ?
Index: [4, 2] value: ?
Index: [0, 3] value: ?
Index: [1, 3] value: ?
Index: [2, 3] value: ?
Index: [3, 3] value: ?
Index: [4, 3] value: ?
Code#
C++#
#include "itkImage.h"
#include "itkImageFileReader.h"
#include "itkImageRegionIteratorWithIndex.h"
#ifdef ENABLE_QUICKVIEW
# include "QuickView.h"
#endif
int
main(int argc, char * argv[])
{
if (argc < 2)
{
std::cerr << "Required: filename" << std::endl;
return EXIT_FAILURE;
}
using ImageType = itk::Image<unsigned char, 2>;
ImageType::Pointer image = itk::ReadImage<ImageType>(argv[1]);
ImageType::SizeType regionSize;
regionSize[0] = 5;
regionSize[1] = 4;
ImageType::IndexType regionIndex;
regionIndex[0] = 0;
regionIndex[1] = 0;
ImageType::RegionType region;
region.SetSize(regionSize);
region.SetIndex(regionIndex);
itk::ImageRegionIteratorWithIndex<ImageType> imageIterator(image, region);
while (!imageIterator.IsAtEnd())
{
std::cout << "Index: " << imageIterator.GetIndex() << " value: " << (int)imageIterator.Get() << std::endl;
// Set the current pixel to white
imageIterator.Set(255);
++imageIterator;
}
#ifdef ENABLE_QUICKVIEW
// Visualize
QuickView viewer;
viewer.AddImage<ImageType>(image);
viewer.Visualize();
#endif
return EXIT_SUCCESS;
}
Classes demonstrated#
-
template<typename TImage>
class ImageRegionIteratorWithIndex : public itk::ImageRegionConstIteratorWithIndex<TImage> A multi-dimensional iterator templated over image type that walks pixels within a region and is specialized to keep track of its image index location.
This class is a specialization of ImageRegionConstIteratorWithIndex that adds write-access (the Set() method). Please see ImageRegionConstIteratorWithIndex for more information.
- MORE INFORMATION
For a complete description of the ITK Image Iterators and their API, please see the Iterators chapter in the ITK Software Guide. The ITK Software Guide is available in print and as a free .pdf download from https://www.itk.org.
- See
ImageConstIterator
- See
ConditionalConstIterator
- See
ConstNeighborhoodIterator
- See
ConstShapedNeighborhoodIterator
- See
ConstSliceIterator
- See
CorrespondenceDataStructureIterator
- See
FloodFilledFunctionConditionalConstIterator
- See
FloodFilledImageFunctionConditionalConstIterator
- See
FloodFilledImageFunctionConditionalIterator
- See
FloodFilledSpatialFunctionConditionalConstIterator
- See
FloodFilledSpatialFunctionConditionalIterator
- See
ImageConstIterator
- See
ImageConstIteratorWithIndex
- See
ImageIterator
- See
ImageIteratorWithIndex
- See
ImageLinearConstIteratorWithIndex
- See
ImageLinearIteratorWithIndex
- See
ImageRandomConstIteratorWithIndex
- See
ImageRandomIteratorWithIndex
- See
ImageRegionConstIterator
- See
ImageRegionConstIteratorWithIndex
- See
ImageRegionExclusionConstIteratorWithIndex
- See
ImageRegionExclusionIteratorWithIndex
- See
ImageRegionIterator
- See
ImageRegionIteratorWithIndex
- See
ImageRegionReverseConstIterator
- See
ImageRegionReverseIterator
- See
ImageReverseConstIterator
- See
ImageReverseIterator
- See
ImageSliceConstIteratorWithIndex
- See
ImageSliceIteratorWithIndex
- See
NeighborhoodIterator
- See
PathConstIterator
- See
PathIterator
- See
ShapedNeighborhoodIterator
- See
SliceIterator
- See
ImageConstIteratorWithIndex
- See
ImageRegionRange
- See
ImageRegionIndexRange
- ITK Sphinx Examples: