Iterate Region in Image With Neighborhood Without Write Access#
Synopsis#
Iterate over a region of an image with a neighborhood (without write access).
Results#
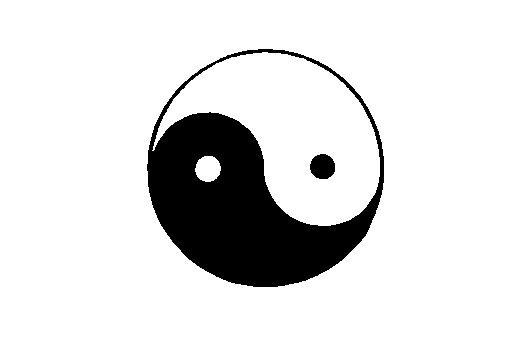
Yinyang.png input image.#
Output:
-1 -1
0 -1
1 -1
-1 0
0 0
1 0
-1 1
0 1
1 1
0 -1
1 -1
2 -1
0 0
1 0
2 0
0 1
1 1
2 1
1 -1
2 -1
3 -1
1 0
2 0
3 0
1 1
2 1
3 1
2 -1
3 -1
4 -1
2 0
3 0
4 0
2 1
3 1
4 1
3 -1
4 -1
5 -1
3 0
4 0
5 0
3 1
4 1
5 1
4 -1
5 -1
6 -1
4 0
5 0
6 0
...
Code#
C++#
#include "itkImage.h"
#include "itkImageFileReader.h"
#include "itkConstNeighborhoodIterator.h"
int
main(int argc, char * argv[])
{
if (argc < 2)
{
std::cerr << "Required: filename" << std::endl;
return EXIT_FAILURE;
}
using ImageType = itk::Image<unsigned char, 2>;
ImageType::Pointer image = itk::ReadImage<ImageType>(argv[1]);
ImageType::SizeType regionSize;
regionSize[0] = 50;
regionSize[1] = 1;
ImageType::IndexType regionIndex;
regionIndex[0] = 0;
regionIndex[1] = 0;
ImageType::RegionType region;
region.SetSize(regionSize);
region.SetIndex(regionIndex);
ImageType::SizeType radius;
radius[0] = 1;
radius[1] = 1;
itk::ConstNeighborhoodIterator<ImageType> iterator(radius, image, region);
while (!iterator.IsAtEnd())
{
for (unsigned int i = 0; i < 9; ++i)
{
ImageType::IndexType index = iterator.GetIndex(i);
std::cout << index[0] << " " << index[1] << std::endl;
bool IsInBounds;
iterator.GetPixel(i, IsInBounds);
}
++iterator;
}
return EXIT_SUCCESS;
}
Classes demonstrated#
-
template<typename TImage, typename TBoundaryCondition = ZeroFluxNeumannBoundaryCondition<TImage>>
class ConstNeighborhoodIterator : public itk::Neighborhood<TImage::InternalPixelType*, TImage::ImageDimension> Const version of NeighborhoodIterator, defining iteration of a local N-dimensional neighborhood of pixels across an itk::Image.
ConstNeighborhoodIterator implements the read-only methods of NeighborhoodIterator. It serves as a base class from which other iterators are derived. See NeighborhoodIterator for more complete information.
- See
Neighborhood
- See
ImageIterator
- See
NeighborhoodIterator
- See
ShapedImageNeighborhoodRange
- ITK Sphinx Examples:
Subclassed by itk::NeighborhoodIterator< TImage, TBoundaryCondition >