Apply a Color Map to an Image#
Synopsis#
Apply a color map to an image
Results#
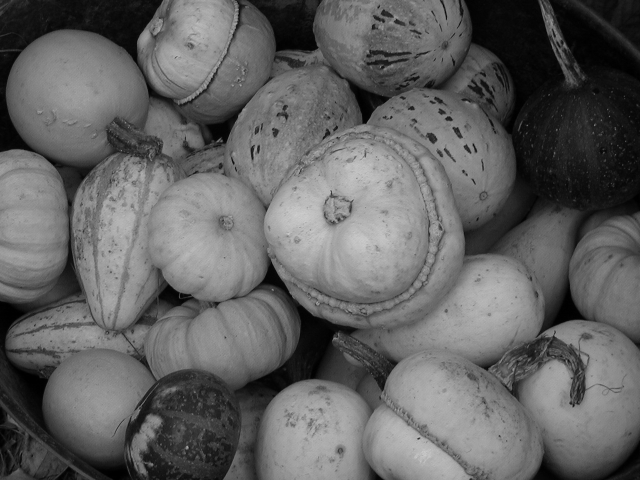
Input image#
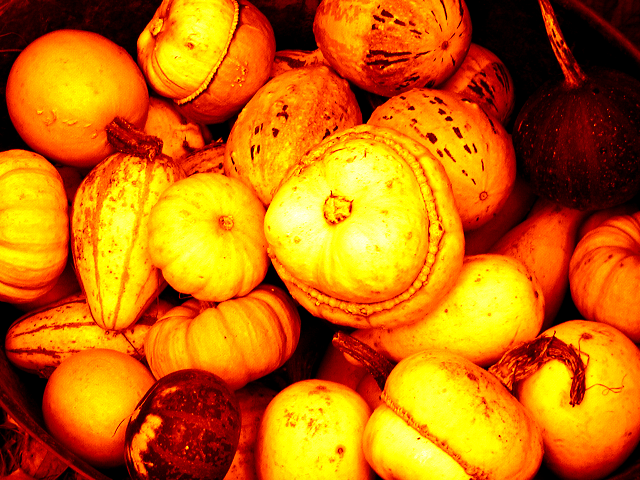
Output image#
Code#
Python#
#!/usr/bin/env python
import itk
import argparse
parser = argparse.ArgumentParser(description="Apply A Colormap To An Image.")
parser.add_argument("input_image")
parser.add_argument("output_image")
args = parser.parse_args()
PixelType = itk.UC
Dimension = 2
ImageType = itk.Image[PixelType, Dimension]
ReaderType = itk.ImageFileReader[ImageType]
reader = ReaderType.New()
reader.SetFileName(args.input_image)
RGBPixelType = itk.RGBPixel[PixelType]
RGBImageType = itk.Image[RGBPixelType, Dimension]
RGBFilterType = itk.ScalarToRGBColormapImageFilter[ImageType, RGBImageType]
rgbfilter = RGBFilterType.New()
rgbfilter.SetInput(reader.GetOutput())
rgbfilter.SetColormap(itk.ScalarToRGBColormapImageFilterEnums.RGBColormapFilter_Hot)
WriterType = itk.ImageFileWriter[RGBImageType]
writer = WriterType.New()
writer.SetFileName(args.output_image)
writer.SetInput(rgbfilter.GetOutput())
writer.Update()
C++#
#include "itkImage.h"
#include "itkImageFileReader.h"
#include "itkImageFileWriter.h"
#include "itkRescaleIntensityImageFilter.h"
#include "itkRGBPixel.h"
#include "itkScalarToRGBColormapImageFilter.h"
int
main(int argc, char * argv[])
{
if (argc != 3)
{
std::cerr << "Usage: " << std::endl;
std::cerr << argv[0];
std::cerr << "<InputFileName> <OutputFileName>";
std::cerr << std::endl;
return EXIT_FAILURE;
}
constexpr unsigned int Dimension = 2;
using PixelType = unsigned char;
using ImageType = itk::Image<PixelType, Dimension>;
const auto input = itk::ReadImage<ImageType>(argv[1]);
using RGBPixelType = itk::RGBPixel<unsigned char>;
using RGBImageType = itk::Image<RGBPixelType, Dimension>;
using RGBFilterType = itk::ScalarToRGBColormapImageFilter<ImageType, RGBImageType>;
auto rgbfilter = RGBFilterType::New();
rgbfilter->SetInput(input);
rgbfilter->SetColormap(itk::ScalarToRGBColormapImageFilterEnums::RGBColormapFilter::Hot);
try
{
itk::WriteImage(rgbfilter->GetOutput(), argv[2]);
}
catch (const itk::ExceptionObject & error)
{
std::cerr << "Error: " << error << std::endl;
return EXIT_FAILURE;
}
return EXIT_SUCCESS;
}
Classes demonstrated#
-
template<typename TInputImage, typename TOutputImage>
class ScalarToRGBColormapImageFilter : public itk::ImageToImageFilter<TInputImage, TOutputImage> Implements pixel-wise intensity->rgb mapping operation on one image.
This class is parameterized over the type of the input image and the type of the output image.
The input image’s scalar pixel values are mapped into a color map. The color map is specified by passing the SetColormap function one of the predefined maps. The following selects the “RGBColormapFilterEnum::Hot” colormap:
RGBFilterType::Pointer colormapImageFilter = RGBFilterType::New(); colormapImageFilter->SetColormap( RGBFilterType::Hot );
You can also specify a custom color map. This is done by creating a CustomColormapFunction, and then creating lists of values for the red, green, and blue channel. An example of setting the red channel of a colormap with only 2 colors is given below. The blue and green channels should be specified in the same manner.
// Create the custom colormap using ColormapType = itk::Function::CustomColormapFunction<RealImageType::PixelType, RGBImageType::PixelType>; ColormapType::Pointer colormap = ColormapType::New(); // Setup the red channel of the colormap ColormapType::ChannelType redChannel; redChannel.push_back(0); redChannel.push_back(255); colormap->SetRedChannel( channel );
The range of values present in the input image is the range that is mapped to the entire range of colors.
This code was contributed in the Insight Journal paper: “Meeting Andy Warhol Somewhere Over the Rainbow: RGB Colormapping and ITK” by Tustison N., Zhang H., Lehmann G., Yushkevich P., Gee J. https://www.insight-journal.org/browse/publication/285
- See
BinaryFunctionImageFilter TernaryFunctionImageFilter
- ITK Sphinx Examples: