Apply Kernel to Every Pixel#
Synopsis#
Apply a kernel to every pixel in an image.
Results#
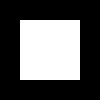
input.png#
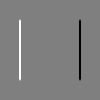
output.png#
Code#
Python#
#!/usr/bin/env python
import sys
import itk
dimension = 2
float_image_type = itk.Image[itk.F, dimension]
unsigned_char_image_type = itk.Image[itk.UC, dimension]
start = itk.Index[dimension]()
start.Fill(0)
size = itk.Size[dimension]()
size.Fill(100)
region = itk.ImageRegion[dimension]()
region.SetIndex(start)
region.SetSize(size)
image = float_image_type.New(Regions=region)
image.Allocate()
image.FillBuffer(0)
image[20:80, 20:80] = 15
input_image = itk.rescale_intensity_image_filter(
image,
output_minimum=0,
output_maximum=255,
ttype=(float_image_type, unsigned_char_image_type),
)
itk.imwrite(input_image, "inputPython.png")
sobel_operator = itk.SobelOperator[itk.F, dimension]()
radius = itk.Size[dimension]()
radius.Fill(1) # a radius of 1x1 creates a 3x3 operator
sobel_operator.SetDirection(0) # Create the operator for the X axis derivative
sobel_operator.CreateToRadius(radius)
image = itk.neighborhood_operator_image_filter(image, operator=sobel_operator)
output_image = itk.rescale_intensity_image_filter(
image,
output_minimum=0,
output_maximum=255,
ttype=(float_image_type, unsigned_char_image_type),
)
itk.imwrite(output_image, "outputPython.png")
C++#
#include "itkImage.h"
#include "itkNeighborhoodOperatorImageFilter.h"
#include "itkSobelOperator.h"
#include "itkImageFileWriter.h"
#include "itkRescaleIntensityImageFilter.h"
using FloatImageType = itk::Image<float, 2>;
void
CreateImage(FloatImageType::Pointer image);
void
CastRescaleAndWrite(FloatImageType::Pointer image, const std::string & filename);
int
main()
{
auto image = FloatImageType::New();
CreateImage(image);
CastRescaleAndWrite(image, "input.png");
using SobelOperatorType = itk::SobelOperator<float, 2>;
SobelOperatorType sobelOperator;
itk::Size<2> radius;
radius.Fill(1); // a radius of 1x1 creates a 3x3 operator
sobelOperator.SetDirection(0); // Create the operator for the X axis derivative
sobelOperator.CreateToRadius(radius);
using NeighborhoodOperatorImageFilterType = itk::NeighborhoodOperatorImageFilter<FloatImageType, FloatImageType>;
auto filter = NeighborhoodOperatorImageFilterType::New();
filter->SetOperator(sobelOperator);
filter->SetInput(image);
filter->Update();
CastRescaleAndWrite(filter->GetOutput(), "output.png");
return EXIT_SUCCESS;
}
void
CreateImage(FloatImageType::Pointer image)
{
FloatImageType::IndexType start;
start.Fill(0);
FloatImageType::SizeType size;
size.Fill(100);
FloatImageType::RegionType region(start, size);
image->SetRegions(region);
image->Allocate();
image->FillBuffer(0);
// Make a square
for (unsigned int r = 20; r < 80; ++r)
{
for (unsigned int c = 20; c < 80; ++c)
{
FloatImageType::IndexType pixelIndex;
pixelIndex[0] = r;
pixelIndex[1] = c;
image->SetPixel(pixelIndex, 15);
}
}
}
void
CastRescaleAndWrite(FloatImageType::Pointer image, const std::string & filename)
{
using UnsignedCharImageType = itk::Image<unsigned char, 2>;
using RescaleFilterType = itk::RescaleIntensityImageFilter<FloatImageType, UnsignedCharImageType>;
auto rescaleFilter = RescaleFilterType::New();
rescaleFilter->SetInput(image);
rescaleFilter->SetOutputMinimum(0);
rescaleFilter->SetOutputMaximum(255);
rescaleFilter->Update();
itk::WriteImage(rescaleFilter->GetOutput(), filename);
}
Classes demonstrated#
-
template<typename TInputImage, typename TOutputImage, typename TOperatorValueType = typename TOutputImage::PixelType>
class NeighborhoodOperatorImageFilter : public itk::ImageToImageFilter<TInputImage, TOutputImage> Applies a single NeighborhoodOperator to an image region.
This filter calculates successive inner products between a single NeighborhoodOperator and a NeighborhoodIterator, which is swept across every pixel in an image region. For operators that are symmetric across their axes, the result is a fast convolution with the image region. Apply the mirror()’d operator for non-symmetric NeighborhoodOperators.
- See
Image
- See
Neighborhood
- See
NeighborhoodOperator
- See
NeighborhoodIterator
- ITK Sphinx Examples:
Subclassed by itk::MaskNeighborhoodOperatorImageFilter< TInputImage, TMaskImage, TOutputImage, TOperatorValueType >, itk::NormalizedCorrelationImageFilter< TInputImage, TMaskImage, TOutputImage, TOperatorValueType >