Extract Given Label Object#
Synopsis#
Extract one given LabelObject from one LabelMap into a new LabelMap and remaining ones into another one.
Results#
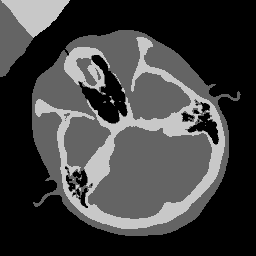
Input image#
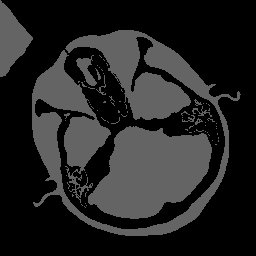
first output (i.e. LabelMap with the LabelObject of interest)#
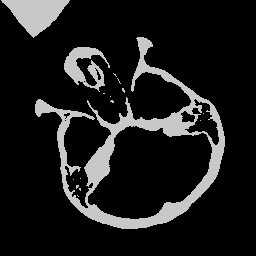
second output (i.e. LabelMap with remaining LabelObjects)#
Code#
C++#
#include "itkImageFileReader.h"
#include "itkImageFileWriter.h"
#include "itkLabelObject.h"
#include "itkLabelMap.h"
#include "itkLabelImageToLabelMapFilter.h"
#include "itkLabelMapToLabelImageFilter.h"
#include "itkLabelSelectionLabelMapFilter.h"
int
main(int argc, char * argv[])
{
if (argc != 5)
{
std::cerr << "Usage: " << std::endl;
std::cerr << argv[0];
std::cerr << " <InputFileName> <OutputFileName1> <OutputFileName2> <Label>";
std::cerr << std::endl;
return EXIT_FAILURE;
}
constexpr unsigned int Dimension = 2;
using PixelType = unsigned char;
using ImageType = itk::Image<PixelType, Dimension>;
const char * inputFileName = argv[1];
const char * outputFileName[2];
outputFileName[0] = argv[2];
outputFileName[1] = argv[3];
const auto label = static_cast<PixelType>(std::stoi(argv[4]));
const auto input = itk::ReadImage<ImageType>(inputFileName);
using LabelObjectType = itk::LabelObject<PixelType, Dimension>;
using LabelMapType = itk::LabelMap<LabelObjectType>;
using LabelImageToLabelMapFilterType = itk::LabelImageToLabelMapFilter<ImageType, LabelMapType>;
auto labelMapConverter = LabelImageToLabelMapFilterType::New();
labelMapConverter->SetInput(input);
labelMapConverter->SetBackgroundValue(itk::NumericTraits<PixelType>::Zero);
using SelectorType = itk::LabelSelectionLabelMapFilter<LabelMapType>;
auto selector = SelectorType::New();
selector->SetInput(labelMapConverter->GetOutput());
selector->SetLabel(label);
for (int i = 0; i < 2; ++i)
{
using LabelMapToLabelImageFilterType = itk::LabelMapToLabelImageFilter<LabelMapType, ImageType>;
auto labelImageConverter = LabelMapToLabelImageFilterType::New();
labelImageConverter->SetInput(selector->GetOutput(i));
try
{
itk::WriteImage(labelImageConverter->GetOutput(), outputFileName[i]);
}
catch (const itk::ExceptionObject & error)
{
std::cerr << "Error: " << error << std::endl;
return EXIT_FAILURE;
}
}
return EXIT_SUCCESS;
}
Classes demonstrated#
-
template<typename TImage>
class LabelSelectionLabelMapFilter : public itk::AttributeSelectionLabelMapFilter<TImage, Functor::LabelLabelObjectAccessor<TImage::LabelObjectType>> remove the objects according to the value of their attribute
LabelSelectionLabelMapFilter removes the objects in a label collection image with an attribute value inside or outside a set of attribute values passed by the user. The attribute is provide by an attribute accessor given in template parameter. Contrary to the other filters made to remove some object of a LabelMap, no ordering relation for the attribute is needed in that filter.
This code was contributed in the Insight Journal paper: “Label object representation and manipulation with ITK” by Lehmann G. https://www.insight-journal.org/browse/publication/176
- Author
Gaetan Lehmann. Biologie du Developpement et de la Reproduction, INRA de Jouy-en-Josas, France.
- See
AttributeLabelObject