Invert Image Using Binary Not Operation#
Synopsis#
Invert an image using the Binary Not operation.
Results#
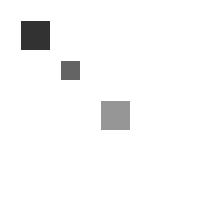
input.png#
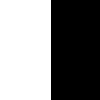
output.png#
Code#
C++#
#include "itkImage.h"
#if ITK_VERSION_MAJOR >= 4
# include "itkBinaryNotImageFilter.h"
#endif
#include "itkImageRegionIterator.h"
#include "itkImageFileWriter.h"
using ImageType = itk::Image<unsigned char, 2>;
static void
CreateImage(ImageType::Pointer image);
int
main()
{
#if ITK_VERSION_MAJOR >= 4
auto image = ImageType::New();
CreateImage(image);
itk::WriteImage(image, "input.png");
using BinaryNotImageFilterType = itk::BinaryNotImageFilter<ImageType>;
auto binaryNotFilter = BinaryNotImageFilterType::New();
binaryNotFilter->SetInput(image);
binaryNotFilter->Update();
itk::WriteImage(binaryNotFilter->GetOutput(), "output.png");
#endif
return EXIT_SUCCESS;
}
void
CreateImage(ImageType::Pointer image)
{
ImageType::IndexType start;
start.Fill(0);
ImageType::SizeType size;
size.Fill(100);
ImageType::RegionType region;
region.SetSize(size);
region.SetIndex(start);
image->SetRegions(region);
image->Allocate();
itk::ImageRegionIterator<ImageType> imageIterator(image, region);
while (!imageIterator.IsAtEnd())
{
if (imageIterator.GetIndex()[0] > 50)
{
imageIterator.Set(255);
}
else
{
imageIterator.Set(0);
}
++imageIterator;
}
}
Classes demonstrated#
-
template<typename TImage>
class BinaryNotImageFilter : public itk::UnaryFunctorImageFilter<TImage, TImage, Functor::BinaryNot<TImage::PixelType>> Implements the BinaryNot logical operator pixel-wise between two images.
This class is parameterized over the types of the two input images and the type of the output image. Numeric conversions (castings) are done by the C++ defaults.
The total operation over one pixel will be
output_pixel = static_cast<PixelType>( input1_pixel != input2_pixel )
Where “!=” is the equality operator in C++.
This implementation was taken from the Insight Journal paper:
https://www.insight-journal.org/browse/publication/176- Author
Gaetan Lehmann. Biologie du Developpement et de la Reproduction, INRA de Jouy-en-Josas, France.
- ITK Sphinx Examples: