Regional Maximal#
Synopsis#
Regional maximal image filter.
Results#
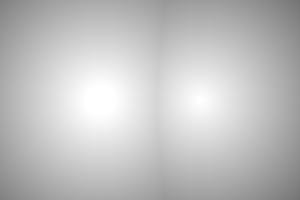
intensityblobs.png#
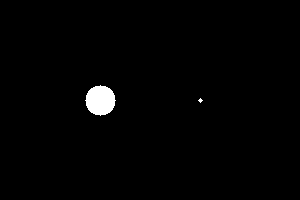
maximal.png#
Code#
C++#
#include "itkImage.h"
#include "itkImageFileWriter.h"
#include "itkRegionalMaximaImageFilter.h"
using ImageType = itk::Image<unsigned char, 2>;
static void
CreateImage(ImageType::Pointer image);
int
main()
{
auto image = ImageType::New();
CreateImage(image);
using RegionalMaximaImageFilter = itk::RegionalMaximaImageFilter<ImageType, ImageType>;
auto filter = RegionalMaximaImageFilter::New();
filter->SetInput(image);
itk::WriteImage(image, "intensityblobs.png");
itk::WriteImage(filter->GetOutput(), "maximal.png");
return EXIT_SUCCESS;
}
void
CreateImage(ImageType::Pointer image)
{
// Create an image with 2 connected components
ImageType::RegionType region;
ImageType::IndexType start;
start[0] = 0;
start[1] = 0;
ImageType::SizeType size;
unsigned int NumRows = 200;
unsigned int NumCols = 300;
size[0] = NumCols;
size[1] = NumRows;
region.SetSize(size);
region.SetIndex(start);
image->SetRegions(region);
image->Allocate();
// Make two intensity blobs
for (unsigned int r = 0; r < NumRows; ++r)
{
for (unsigned int c = 0; c < NumCols; ++c)
{
ImageType::IndexType pixelIndex;
pixelIndex[0] = c;
pixelIndex[1] = r;
double c1 = c - 100.0;
double c2 = c - 200.0;
double rr = r - 100.0;
// purposely use 270,257 since it is > 255
double v1 = 270.0 - std::sqrt(rr * rr + c1 * c1);
double v2 = 257.0 - std::sqrt(rr * rr + c2 * c2);
double maxv = v1;
if (maxv < v2)
maxv = v2;
double val = maxv;
if (val < 0.0)
val = 0.0;
if (val > 255.0)
val = 255.0;
image->SetPixel(pixelIndex, val);
}
}
}
Classes demonstrated#
-
template<typename TInputImage, typename TOutputImage>
class RegionalMaximaImageFilter : public itk::ImageToImageFilter<TInputImage, TOutputImage> Produce a binary image where foreground is the regional maxima of the input image.
Regional maxima are flat zones surrounded by pixels of lower value.
If the input image is constant, the entire image can be considered as a maxima or not. The desired behavior can be selected with the SetFlatIsMaxima() method.
This class was contributed to the Insight Journal by author Gaetan Lehmann. Biologie du Developpement et de la Reproduction, INRA de Jouy-en-Josas, France. The paper can be found at
https://www.insight-journal.org/browse/publication/65- Author
Gaetan Lehmann
- See
ValuedRegionalMaximaImageFilter
- See
HConvexImageFilter
- See
RegionalMinimaImageFilter
- ITK Sphinx Examples: