Threshold an Image#
Synopsis#
Threshold an image using itk::ThresholdImageFilter
Results#
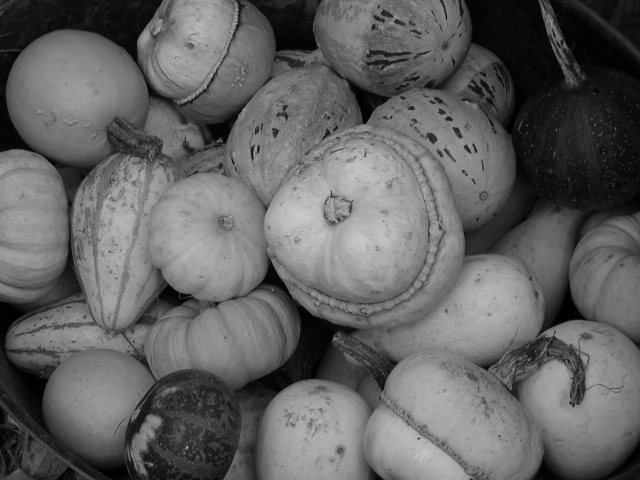
Input image#
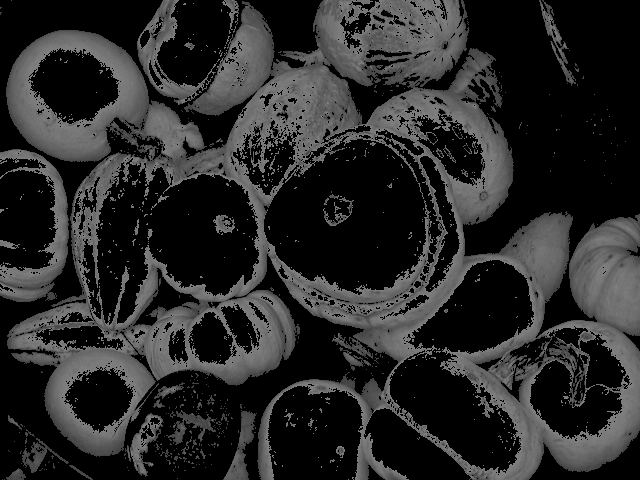
Output image#
Code#
Python#
#!/usr/bin/env python
import itk
import argparse
parser = argparse.ArgumentParser(description="Threshold An Image.")
parser.add_argument("input_image")
parser.add_argument("output_image")
parser.add_argument("lower_threshold", type=int)
parser.add_argument("upper_threshold", type=int)
args = parser.parse_args()
PixelType = itk.UC
Dimension = 2
ImageType = itk.Image[PixelType, Dimension]
reader = itk.ImageFileReader[ImageType].New()
reader.SetFileName(args.input_image)
thresholdFilter = itk.ThresholdImageFilter[ImageType].New()
thresholdFilter.SetInput(reader.GetOutput())
thresholdFilter.ThresholdOutside(args.lower_threshold, args.upper_threshold)
thresholdFilter.SetOutsideValue(0)
writer = itk.ImageFileWriter[ImageType].New()
writer.SetFileName(args.output_image)
writer.SetInput(thresholdFilter.GetOutput())
writer.Update()
C++#
#include "itkImageFileReader.h"
#include "itkImageFileWriter.h"
#include "itkThresholdImageFilter.h"
int
main(int argc, char * argv[])
{
if (argc != 5)
{
std::cerr << "Usage: " << std::endl;
std::cerr << argv[0];
std::cerr << " <InputFileName> <OutputFileName> <Lower Threshold> <Upper Threshold>";
std::cerr << std::endl;
return EXIT_FAILURE;
}
const char * inputFileName = argv[1];
const char * outputFileName = argv[2];
constexpr unsigned int Dimension = 2;
using PixelType = unsigned char;
using ImageType = itk::Image<PixelType, Dimension>;
const auto input = itk::ReadImage<ImageType>(inputFileName);
unsigned char lowerThreshold = std::stoi(argv[3]);
unsigned char upperThreshold = std::stoi(argv[4]);
using FilterType = itk::ThresholdImageFilter<ImageType>;
auto filter = FilterType::New();
filter->SetInput(input);
filter->ThresholdOutside(lowerThreshold, upperThreshold);
filter->SetOutsideValue(0);
try
{
itk::WriteImage(filter->GetOutput(), outputFileName);
}
catch (const itk::ExceptionObject & error)
{
std::cerr << "Error: " << error << std::endl;
return EXIT_FAILURE;
}
return EXIT_SUCCESS;
}
Classes demonstrated#
-
template<typename TImage>
class ThresholdImageFilter : public itk::InPlaceImageFilter<TImage, TImage> Set image values to a user-specified value if they are below, above, or between simple threshold values.
ThresholdImageFilter sets image values to a user-specified “outside” value (by default, “black”) if the image values are below, above, or between simple threshold values.
The available methods are:
ThresholdAbove(): The values greater than the threshold value are set to OutsideValue
ThresholdBelow(): The values less than the threshold value are set to OutsideValue
ThresholdOutside(): The values outside the threshold range (less than lower or greater than upper) are set to OutsideValue
Note that these definitions indicate that pixels equal to the threshold value are not set to OutsideValue in any of these methods
The pixels must support the operators >= and <=.
- ITK Sphinx Examples: