Create Triangular Quad Edge Mesh#
Synopsis#
Create a triangular surface mesh using itk::QuadEdgeMesh
Results#
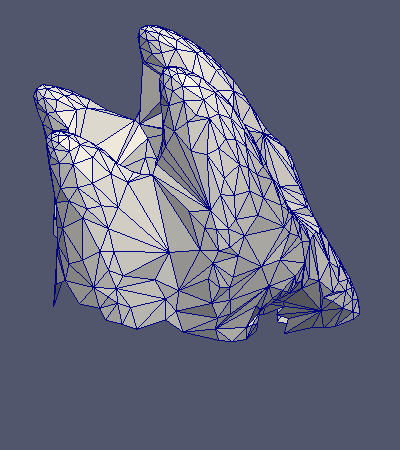
Output mesh#
Code#
C++#
#include "itkQuadEdgeMesh.h"
#include "itkMeshFileWriter.h"
int
main(int argc, char * argv[])
{
if (argc != 2)
{
std::cerr << "Usage: " << std::endl;
std::cerr << argv[0];
std::cerr << " <OutputFileName>";
std::cerr << std::endl;
return EXIT_FAILURE;
}
const char * outputFileName = argv[1];
constexpr unsigned int Dimension = 3;
using CoordType = double;
using MeshType = itk::QuadEdgeMesh<CoordType, Dimension>;
auto mesh = MeshType::New();
using PointsContainer = MeshType::PointsContainer;
using PointsContainerPointer = MeshType::PointsContainerPointer;
PointsContainerPointer points = PointsContainer::New();
points->Reserve(100);
using PointType = MeshType::PointType;
PointType p;
p[2] = 0.;
using PointIdentifier = MeshType::PointIdentifier;
PointIdentifier k = 0;
for (int i = 0; i < 10; ++i)
{
p[0] = static_cast<CoordType>(i);
for (int j = 0; j < 10; ++j)
{
p[1] = static_cast<CoordType>(j);
points->SetElement(k, p);
k++;
}
}
mesh->SetPoints(points);
k = 0;
for (int i = 0; i < 9; ++i)
{
for (int j = 0; j < 9; ++j)
{
mesh->AddFaceTriangle(k, k + 1, k + 11);
mesh->AddFaceTriangle(k, k + 11, k + 10);
k++;
}
k++;
}
using WriterType = itk::MeshFileWriter<MeshType>;
auto writer = WriterType::New();
writer->SetFileName(outputFileName);
writer->SetInput(mesh);
try
{
writer->Update();
}
catch (const itk::ExceptionObject & error)
{
std::cerr << "Error: " << error << std::endl;
return EXIT_FAILURE;
}
return EXIT_SUCCESS;
}
Classes demonstrated#
-
template<typename TPixel, unsigned int VDimension, typename TTraits = QuadEdgeMeshTraits<TPixel, VDimension, bool, bool>>
class QuadEdgeMesh : public itk::Mesh<TPixel, VDimension, TTraits> Mesh class for 2D manifolds embedded in ND space.
This implementation was contributed as a paper to the Insight Journal
https://www.insight-journal.org/browse/publication/122- Author
Alexandre Gouaillard, Leonardo Florez-Valencia, Eric Boix