Add Noise To Binary Image#
Synopsis#
Add noise to a binary image.
Results#
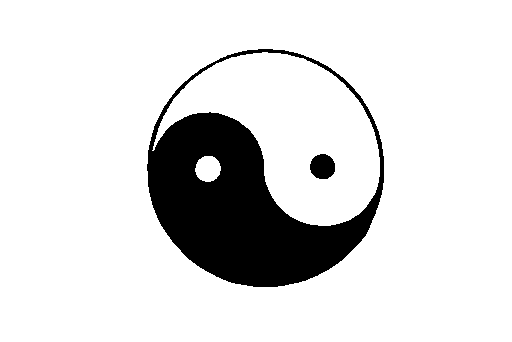
Yinyang.png#
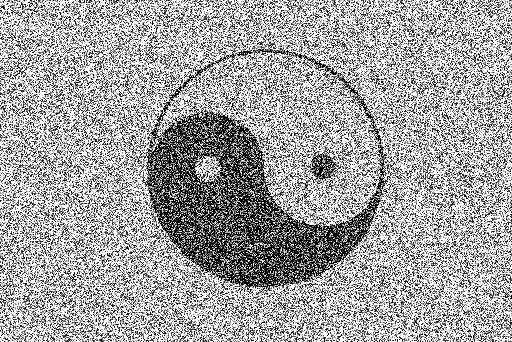
Output.png#
Output:
Number of random samples: 105062
Code#
C++#
#include "itkImage.h"
#include "itkImageFileReader.h"
#include "itkImageRandomNonRepeatingIteratorWithIndex.h"
#include "itkImageFileWriter.h"
#include "itkMersenneTwisterRandomVariateGenerator.h"
int
main(int argc, char * argv[])
{
if (argc < 3)
{
std::cerr << "Usage: " << argv[0] << " inputFile outputFile [percent]" << std::endl;
return EXIT_FAILURE;
}
double percent = .1;
if (argc > 3)
{
percent = std::stod(argv[3]);
if (percent >= 1.0)
{
percent /= 100.0;
}
}
using ImageType = itk::Image<unsigned char, 2>;
using IteratorType = itk::ImageRandomNonRepeatingIteratorWithIndex<ImageType>;
const auto input = itk::ReadImage<ImageType>(argv[1]);
// At x% of the pixels, add a uniform random value between 0 and 255
IteratorType it(input, input->GetLargestPossibleRegion());
it.SetNumberOfSamples(input->GetLargestPossibleRegion().GetNumberOfPixels() * percent);
std::cout << "Number of random samples: " << it.GetNumberOfSamples() << std::endl;
using GeneratorType = itk::Statistics::MersenneTwisterRandomVariateGenerator;
auto random = GeneratorType::New();
it.GoToBegin();
while (!it.IsAtEnd())
{
it.Set(random->GetUniformVariate(0, 255));
++it;
}
itk::WriteImage(input, argv[2]);
return EXIT_SUCCESS;
}
Classes demonstrated#
-
template<typename TImage>
class ImageRandomNonRepeatingIteratorWithIndex : public itk::ImageRandomNonRepeatingConstIteratorWithIndex<TImage> A multi-dimensional image iterator that visits image pixels within a region in a random order, without repeating.
This class was contributed by Rupert Brooks, McGill Centre for Intelligent Machines, Montreal, Canada. It is heavily based on the ImageRandomIterator class.
This iterator is a subclass of itk::ImageRandomNonRepeatingConstIteratorWithIndex that adds write-access functionality. Please see itk::ImageRandomNonRepeatingConstIteratorWithIndex for more information.
- MORE INFORMATION
For a complete description of the ITK Image Iterators and their API, please see the Iterators chapter in the ITK Software Guide. The ITK Software Guide is available in print and as a free .pdf download from https://www.itk.org.
- Author
Rupert Brooks, McGill Centre for Intelligent Machines. Canada
- See
ImageConstIterator
- See
ConditionalConstIterator
- See
ConstNeighborhoodIterator
- See
ConstShapedNeighborhoodIterator
- See
ConstSliceIterator
- See
CorrespondenceDataStructureIterator
- See
FloodFilledFunctionConditionalConstIterator
- See
FloodFilledImageFunctionConditionalConstIterator
- See
FloodFilledImageFunctionConditionalIterator
- See
FloodFilledSpatialFunctionConditionalConstIterator
- See
FloodFilledSpatialFunctionConditionalIterator
- See
ImageConstIterator
- See
ImageConstIteratorWithIndex
- See
ImageIterator
- See
ImageIteratorWithIndex
- See
ImageRandomNonRepeatingConstIteratorWithIndex
- See
ImageRandomNonRepeatingIteratorWithIndex
- See
ImageRandomConstIteratorWithIndex
- See
ImageRandomIteratorWithIndex
- See
ImageRegionConstIterator
- See
ImageRegionConstIteratorWithIndex
- See
ImageRegionExclusionConstIteratorWithIndex
- See
ImageRegionExclusionIteratorWithIndex
- See
ImageRegionIterator
- See
ImageRegionIteratorWithIndex
- See
ImageRegionReverseConstIterator
- See
ImageRegionReverseIterator
- See
ImageReverseConstIterator
- See
ImageReverseIterator
- See
ImageSliceConstIteratorWithIndex
- See
ImageSliceIteratorWithIndex
- See
NeighborhoodIterator
- See
PathConstIterator
- See
PathIterator
- See
ShapedNeighborhoodIterator
- See
SliceIterator
- See
ImageConstIteratorWithIndex
- ITK Sphinx Examples: