In Place Filter of Image#
Synopsis#
In-place filtering of an image.
Results#
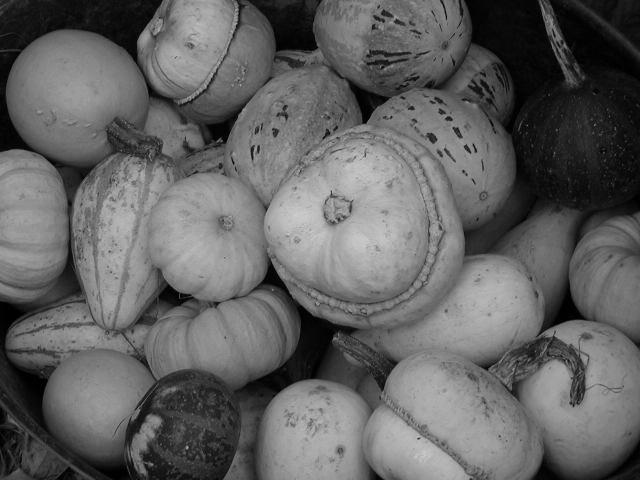
Input Image#
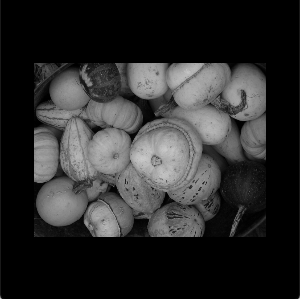
Output In QuickView#
Code#
C++#
#include "itkImage.h"
#include "itkImageFileReader.h"
#include "itkBinaryThresholdImageFilter.h"
#include <itkImageToVTKImageFilter.h>
#include "vtkVersion.h"
#include "vtkSmartPointer.h"
#include "vtkRenderWindow.h"
#include "vtkRenderer.h"
#include "vtkInteractorStyleImage.h"
#include "vtkRenderWindowInteractor.h"
#include "vtkImageActor.h"
#include "vtkImageMapper3D.h"
#include "vtkImageViewer.h"
using ImageType = itk::Image<unsigned char, 2>;
static void
ApplyThresholding(ImageType::Pointer image);
int
main(int argc, char * argv[])
{
if (argc < 2)
{
std::cerr << "Required: filename" << std::endl;
return EXIT_FAILURE;
}
ImageType::Pointer image = itk::ReadImage<ImageType>(argv[1]);
ApplyThresholding(image);
// Visualize
using ConnectorType = itk::ImageToVTKImageFilter<ImageType>;
auto connector = ConnectorType::New();
connector->SetInput(image);
vtkSmartPointer<vtkImageActor> actor = vtkSmartPointer<vtkImageActor>::New();
#if VTK_MAJOR_VERSION <= 5
actor->SetInput(connector->GetOutput());
#else
connector->Update();
actor->GetMapper()->SetInputData(connector->GetOutput());
#endif
// There will be one render window
vtkSmartPointer<vtkRenderWindow> renderWindow = vtkSmartPointer<vtkRenderWindow>::New();
vtkSmartPointer<vtkRenderWindowInteractor> interactor = vtkSmartPointer<vtkRenderWindowInteractor>::New();
interactor->SetRenderWindow(renderWindow);
vtkSmartPointer<vtkRenderer> renderer = vtkSmartPointer<vtkRenderer>::New();
renderWindow->AddRenderer(renderer);
renderer->AddActor(actor);
renderer->ResetCamera();
renderWindow->Render();
vtkSmartPointer<vtkInteractorStyleImage> style = vtkSmartPointer<vtkInteractorStyleImage>::New();
interactor->SetInteractorStyle(style);
interactor->Start();
return EXIT_SUCCESS;
}
void
ApplyThresholding(ImageType::Pointer image)
{
using BinaryThresholdImageFilterType = itk::BinaryThresholdImageFilter<ImageType, ImageType>;
auto thresholdFilter = BinaryThresholdImageFilterType::New();
thresholdFilter->SetInput(image);
thresholdFilter->SetLowerThreshold(10);
thresholdFilter->SetUpperThreshold(50);
thresholdFilter->SetInsideValue(255);
thresholdFilter->SetOutsideValue(0);
thresholdFilter->InPlaceOn();
thresholdFilter->Update();
}
Classes demonstrated#
-
template<typename TInputImage, typename TOutputImage = TInputImage>
class InPlaceImageFilter : public itk::ImageToImageFilter<TInputImage, TOutputImage> Base class for filters that take an image as input and overwrite that image as the output.
InPlaceImageFilter is the base class for all process objects whose output image data is constructed by overwriting the input image data. In other words, the output bulk data is the same block of memory as the input bulk data. This filter provides the mechanisms for in place image processing while maintaining general pipeline mechanics. InPlaceImageFilters use less memory than standard ImageToImageFilters because the input buffer is reused as the output buffer. However, this benefit does not come without a cost. Since the filter overwrites its input, the ownership of the bulk data is transitioned from the input data object to the output data object. When a data object has multiple consumers with one of the consumers being an in place filter, the in place filter effectively destroys the bulk data for the data object. Upstream filters will then have to re-execute to regenerate the data object’s bulk data for the remaining consumers.
Since an InPlaceImageFilter reuses the input bulk data memory for the output bulk data memory, the input image type must match the output image type. If the input and output image types are not identical, the filter reverts to a traditional ImageToImageFilter behaviour where an output image is allocated. Additionally, the requested region of the output must match that of the input. In place operation can also be controlled (when the input and output image type match) via the methods InPlaceOn() and InPlaceOff().
Subclasses of InPlaceImageFilter must take extra care in how they manage memory using (and perhaps overriding) the implementations of ReleaseInputs() and AllocateOutputs() provided here.
- ITK Sphinx Examples:
Subclassed by itk::BinaryGeneratorImageFilter< TInputImage, TLabelImage, TOutputImage >, itk::BinaryGeneratorImageFilter< TInputImage, TMaskImage, TOutputImage >, itk::BinaryContourImageFilter< TInputImage, TOutputImage >, itk::CastImageFilter< TInputImage, TOutputImage >, itk::ExtractImageFilter< TInputImage, TOutputImage >, itk::FiniteDifferenceImageFilter< TInputImage, TOutputImage >, itk::GradientMagnitudeRecursiveGaussianImageFilter< TInputImage, TOutputImage >, itk::LabelContourImageFilter< TInputImage, TOutputImage >, itk::NaryFunctorImageFilter< TInputImage, TOutputImage, TFunction >, itk::NoiseBaseImageFilter< TInputImage, TOutputImage >, itk::PasteImageFilter< TInputImage, TSourceImage, TOutputImage >, itk::RecursiveSeparableImageFilter< TInputImage, TOutputImage >, itk::RelabelComponentImageFilter< TInputImage, TOutputImage >, itk::SmoothingRecursiveGaussianImageFilter< TInputImage, TOutputImage >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, TFunction >, itk::UnaryGeneratorImageFilter< TInputImage, TOutputImage >, itk::NaryFunctorImageFilter< TInputImage, TOutputImage, Functor::Add1< TInputImage::PixelType, TInputImage::PixelType > >, itk::NaryFunctorImageFilter< TInputImage, TOutputImage, Functor::Maximum1< TInputImage::PixelType, TInputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::AccessorFunctor< TInputImage::PixelType, TAccessor > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::BinaryThreshold< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::ChangeLabel< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::Clamp< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::ExpNegative< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::IntensityLinearTransform< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::IntensityWindowingTransform< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::InvertIntensityTransform< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::MatrixIndexSelection< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::NOT< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::Sigmoid< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::SymmetricEigenAnalysisFixedDimensionFunction< TMatrixDimension, TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::SymmetricEigenAnalysisFunction< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::TensorFractionalAnisotropyFunction< TInputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::TensorRelativeAnisotropyFunction< TInputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::ThresholdLabeler< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::VectorIndexSelectionCast< TInputImage::PixelType, TOutputImage::PixelType > >, itk::UnaryFunctorImageFilter< TInputImage, TOutputImage, Functor::VectorMagnitudeLinearTransform< TInputImage::PixelType, TOutputImage::PixelType > >