Iterate Line Through Image#
Synopsis#
Iterate over a line through an image.
Results#
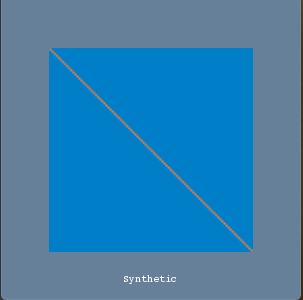
Output In VTK Window#
Code#
C++#
#include "itkImage.h"
#include "itkRGBPixel.h"
#include "itkImageFileReader.h"
#include "itkImageRegionIterator.h"
#include "itkLineIterator.h"
#include "itksys/SystemTools.hxx"
#ifdef ENABLE_QUICKVIEW
# include "QuickView.h"
#endif
using PixelType = itk::RGBPixel<unsigned char>;
using ImageType = itk::Image<PixelType, 2>;
static void
CreateImage(ImageType::Pointer image);
int
main(int argc, char * argv[])
{
auto image = ImageType::New();
std::string inputFilename;
if (argc < 2)
{
CreateImage(image);
inputFilename = "Synthetic";
}
else
{
image = itk::ReadImage<ImageType>(argv[1]);
inputFilename = argv[1];
}
PixelType pixel;
pixel.SetRed(255);
pixel.SetGreen(127);
pixel.SetBlue(50);
ImageType::RegionType region = image->GetLargestPossibleRegion();
ImageType::IndexType corner1 = region.GetIndex();
ImageType::IndexType corner2;
corner2[0] = corner1[0] + region.GetSize()[0] - 1;
corner2[1] = corner1[1] + region.GetSize()[1] - 1;
itk::LineIterator<ImageType> it1(image, corner1, corner2);
it1.GoToBegin();
while (!it1.IsAtEnd())
{
it1.Set(pixel);
++it1;
}
#ifdef ENABLE_QUICKVIEW
QuickView viewer;
if (argc > 1)
{
viewer.AddImage<ImageType>(image, true, itksys::SystemTools::GetFilenameName(argv[1]));
}
else
{
viewer.AddImage<ImageType>(image, true, "Synthetic");
}
viewer.Visualize();
#endif
return EXIT_SUCCESS;
}
void
CreateImage(ImageType::Pointer image)
{
ImageType::SizeType regionSize;
regionSize.Fill(100);
ImageType::IndexType regionIndex;
regionIndex.Fill(0);
ImageType::RegionType region;
region.SetSize(regionSize);
region.SetIndex(regionIndex);
PixelType pixel;
pixel.SetRed(0);
pixel.SetGreen(127);
pixel.SetBlue(200);
image->SetRegions(region);
image->Allocate();
image->FillBuffer(pixel);
}
Classes demonstrated#
-
template<typename TImage>
class LineIterator : public itk::LineConstIterator<TImage> An iterator that walks a Bresenham line through an ND image with write access to pixels.
LineIterator is an iterator that walks a Bresenham line through an image. The iterator is constructed similar to other image iterators, except instead of specifying a region to traverse, you specify two indices. The interval specified by the two indices is closed. So, a line iterator specified with the same start and end index will visit exactly one pixel.
LineConstIterator<ImageType> it(image, I1, I2); while (!it.IsAtEnd()) { // visits at least 1 pixel }
- Author
Benjamin King, Experimentelle Radiologie, Medizinische Hochschule Hannover.
- See
LineConstIterator
- ITK Sphinx Examples: