Compute Local Noise in Image#
Synopsis#
Compute the local noise in an image.
Results#
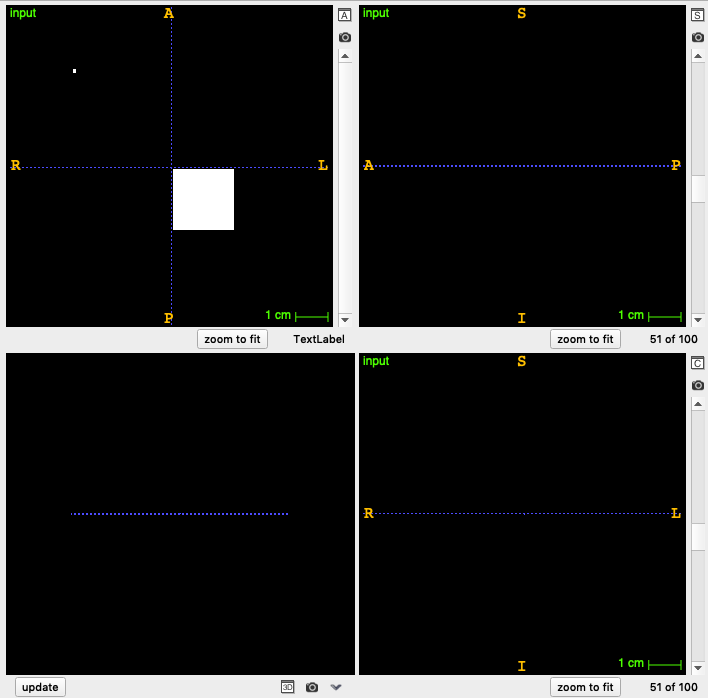
input.mhd#
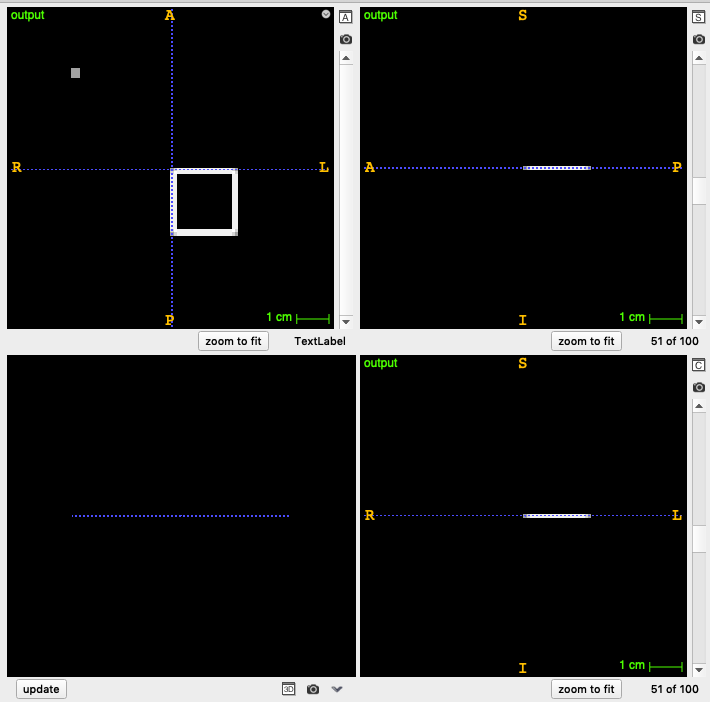
output.mhd#
Code#
C++#
#include "itkImage.h"
#include "itkNoiseImageFilter.h"
#include "itkImageFileWriter.h"
using ImageType = itk::Image<float, 2>;
void
CreateImage(ImageType::Pointer image);
int
main(int itkNotUsed(argc), char * itkNotUsed(argv)[])
{
auto image = ImageType::New();
CreateImage(image);
using NoiseImageFilterType = itk::NoiseImageFilter<ImageType, ImageType>;
auto noiseImageFilter = NoiseImageFilterType::New();
noiseImageFilter->SetInput(image);
noiseImageFilter->SetRadius(1);
noiseImageFilter->Update();
itk::WriteImage(noiseImageFilter->GetOutput(), "output.mhd");
return EXIT_SUCCESS;
}
void
CreateImage(ImageType::Pointer image)
{
// Create an image that is mostly constant but has some different kinds of objects.
ImageType::IndexType start;
start.Fill(0);
ImageType::SizeType size;
size.Fill(100);
ImageType::RegionType region(start, size);
// Create a black image
image->SetRegions(region);
image->Allocate();
image->FillBuffer(0);
// Create a white square
itk::ImageRegionIterator<ImageType> imageIterator(image, region);
while (!imageIterator.IsAtEnd())
{
if (imageIterator.GetIndex()[0] > 50 && imageIterator.GetIndex()[0] < 70 && imageIterator.GetIndex()[1] > 50 &&
imageIterator.GetIndex()[1] < 70)
{
imageIterator.Set(255);
}
++imageIterator;
}
// Create a rogue white pixel
ImageType::IndexType pixel;
pixel.Fill(20);
image->SetPixel(pixel, 255);
itk::WriteImage(image, "input.mhd");
}
Classes demonstrated#
-
template<typename TInputImage, typename TOutputImage>
class NoiseImageFilter : public itk::BoxImageFilter<TInputImage, TOutputImage> Calculate the local noise in an image.
Computes an image where a given pixel is the standard deviation of the pixels in a neighborhood about the corresponding input pixel. This serves as an estimate of the local noise (or texture) in an image. Currently, this noise estimate assume a piecewise constant image. This filter should be extended to fitting a (hyper) plane to the neighborhood and calculating the standard deviation of the residuals to this (hyper) plane.
- See
Image
- See
Neighborhood
- See
NeighborhoodOperator
- See
NeighborhoodIterator
- ITK Sphinx Examples: