Pad Image by Wrapping#
Synopsis#
Pad an image by wrapping.
Results#
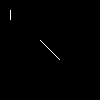
image.png#
Code#
C++#
#include "itkImage.h"
#include "itkRescaleIntensityImageFilter.h"
#include "itkWrapPadImageFilter.h"
#include "itkImageRegionIterator.h"
using ImageType = itk::Image<unsigned char, 2>;
static void
CreateImage(ImageType::Pointer image);
int
main()
{
auto image = ImageType::New();
CreateImage(image);
using WrapPadImageFilterType = itk::WrapPadImageFilter<ImageType, ImageType>;
ImageType::SizeType lowerBound;
lowerBound[0] = 20;
lowerBound[1] = 30;
ImageType::SizeType upperBound;
upperBound[0] = 50;
upperBound[1] = 40;
auto padFilter = WrapPadImageFilterType::New();
padFilter->SetInput(image);
padFilter->SetPadLowerBound(lowerBound);
padFilter->SetPadUpperBound(upperBound);
padFilter->Update();
return EXIT_SUCCESS;
}
void
CreateImage(ImageType::Pointer image)
{
// Create an image
ImageType::RegionType region;
ImageType::IndexType start;
start[0] = 0;
start[1] = 0;
ImageType::SizeType size;
size[0] = 200;
size[1] = 100;
region.SetSize(size);
region.SetIndex(start);
image->SetRegions(region);
image->Allocate();
itk::ImageRegionIterator<ImageType> imageIterator(image, image->GetLargestPossibleRegion());
while (!imageIterator.IsAtEnd())
{
imageIterator.Set(imageIterator.GetIndex()[0]);
++imageIterator;
}
}
Classes demonstrated#
-
template<typename TInputImage, typename TOutputImage>
class WrapPadImageFilter : public itk::PadImageFilter<TInputImage, TOutputImage> Increase the image size by padding with replicants of the input image value.
WrapPadImageFilter changes the image bounds of an image. Added pixels are filled in with a wrapped replica of the input image. For instance, if the output image needs a pixel that is two pixels to the left of the LargestPossibleRegion of the input image, the value assigned will be from the pixel two pixels inside the right boundary of the LargestPossibleRegion. The image bounds of the output must be specified.
This filter is implemented as a multithreaded filter. It provides a ThreadedGenerateData() method for its implementation.
- See
MirrorPadImageFilter, ConstantPadImageFilter
- ITK Sphinx Examples: